Getting Started With MongoDB and FastAPI
Aaron Bassett, Mark Smith7 min read • Published Feb 05, 2022 • Updated Jul 12, 2024
Rate this quickstart
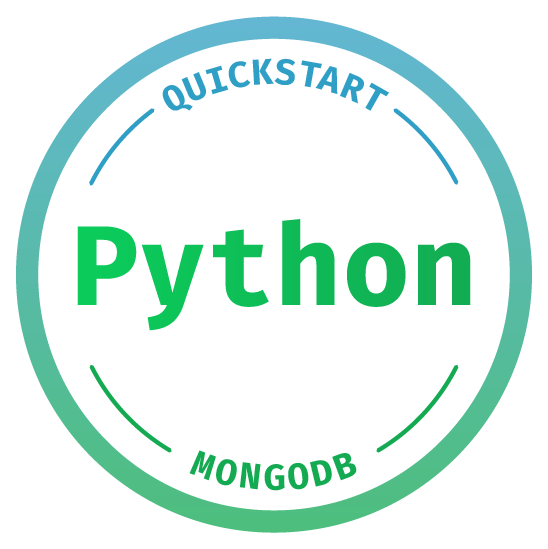
FastAPI is a modern, high-performance, easy-to-learn, fast-to-code, production-ready, Python 3.6+ framework for building APIs based on standard Python type hints. While it might not be as established as some other Python frameworks such as Django, it is already in production at companies such as Uber, Netflix, and Microsoft.
FastAPI is async, and as its name implies, it is super fast; so, MongoDB is the perfect accompaniment. In this quick start, we will create a CRUD (Create, Read, Update, Delete) app showing how you can integrate MongoDB with your FastAPI projects.
- Python 3.9.0
- A MongoDB Atlas cluster. Follow the "Get Started with Atlas" guide to create your account and MongoDB cluster. Keep a note of your username, password, and connection string as you will need those later.
1 git clone git@github.com:mongodb-developer/mongodb-with-fastapi.git
You will need to install a few dependencies: FastAPI, Motor, etc. I always recommend that you install all Python dependencies in a virtualenv for the project. Before running pip, ensure your virtualenv is active.
1 cd mongodb-with-fastapi 2 pip install -r requirements.txt
It may take a few moments to download and install your dependencies. This is normal, especially if you have not installed a particular package before.
Once you have installed the dependencies, you need to create an environment variable for your MongoDB connection string.
1 export MONGODB_URL="mongodb+srv://<username>:<password>@<url>/<db>?retryWrites=true&w=majority"
Remember, anytime you start a new terminal session, you will need to set this environment variable again. I use direnv to make this process easier.
The final step is to start your FastAPI server.
1 uvicorn app:app --reload
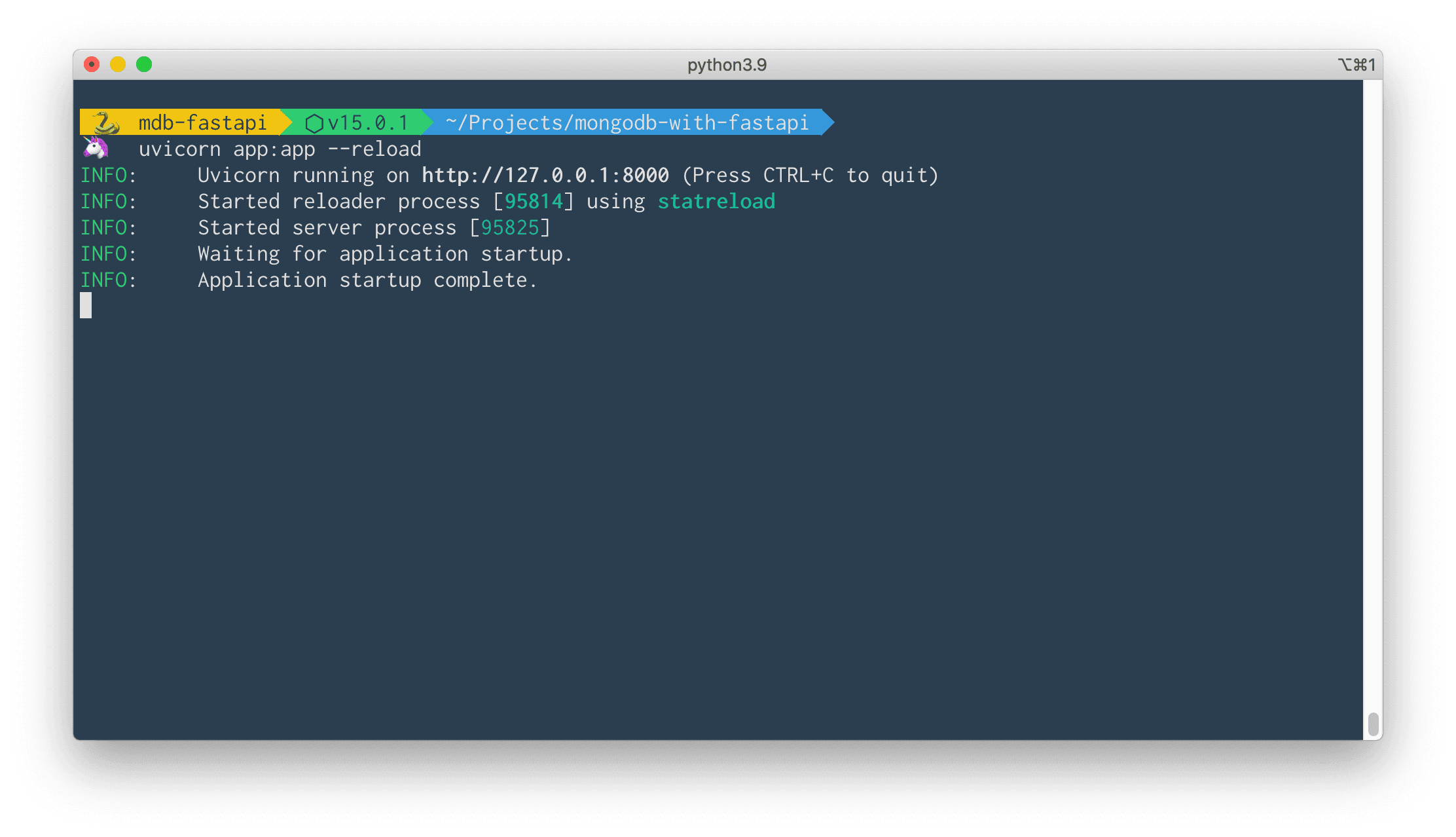
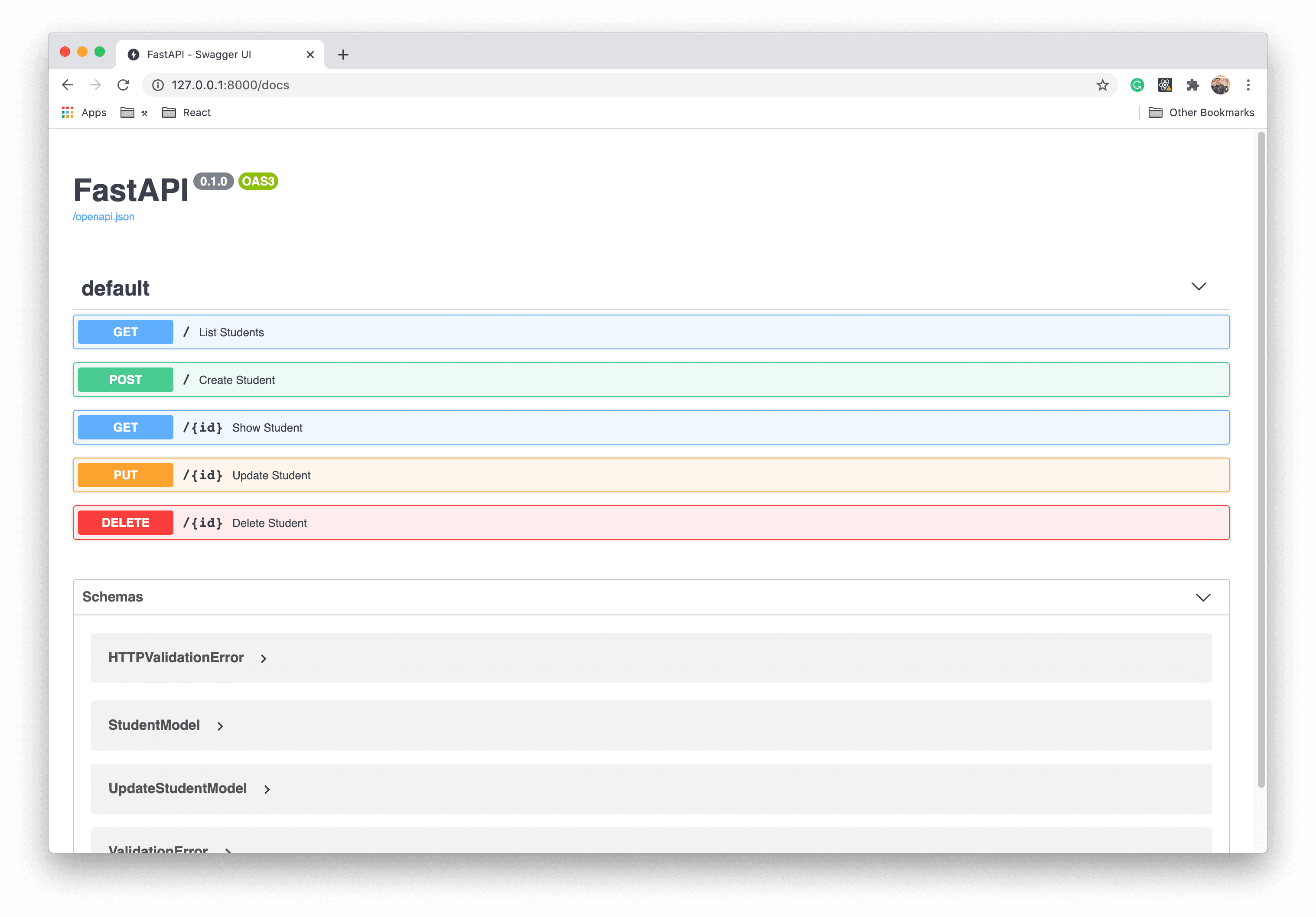
Once you have had a chance to try the example, come back and we will walk through the code.
All the code for the example application is within
app.py
. I'll break it down into sections and walk through what each is doing.One of the very first things we do is connect to our MongoDB database.
1 client = motor.motor_asyncio.AsyncIOMotorClient(os.environ["MONGODB_URL"]) 2 db = client.get_database("college") 3 student_collection = db.get_collection("students")
We're using the async motor driver to create our MongoDB client, and then we specify our database name
college
.1 # Represents an ObjectId field in the database. 2 # It will be represented as a `str` on the model so that it can be serialized to JSON. 3 PyObjectId = Annotated[str, BeforeValidator(str)]
MongoDB stores data as BSON. FastAPI encodes and decodes data as JSON strings. BSON has support for additional non-JSON-native data types, including
ObjectId
which can't be directly encoded as JSON. Because of this, we convert ObjectId
s to strings before storing them as the id
field.Many people think of MongoDB as being schema-less, which is wrong. MongoDB has a flexible schema. That is to say that collections do not enforce document structure by default, so you have the flexibility to make whatever data-modelling choices best match your application and its performance requirements. So, it's not unusual to create models when working with a MongoDB database. Our application has three models, the
StudentModel
, the UpdateStudentModel
, and the StudentCollection
.1 class StudentModel(BaseModel): 2 """ 3 Container for a single student record. 4 """ 5 6 # The primary key for the StudentModel, stored as a `str` on the instance. 7 # This will be aliased to `_id` when sent to MongoDB, 8 # but provided as `id` in the API requests and responses. 9 id: Optional[PyObjectId] = Field(alias="_id", default=None) 10 name: str = Field(...) 11 email: EmailStr = Field(...) 12 course: str = Field(...) 13 gpa: float = Field(..., le=4.0) 14 model_config = ConfigDict( 15 populate_by_name=True, 16 arbitrary_types_allowed=True, 17 json_schema_extra={ 18 "example": { 19 "name": "Jane Doe", 20 "email": "jdoe@example.com", 21 "course": "Experiments, Science, and Fashion in Nanophotonics", 22 "gpa": 3.0, 23 } 24 }, 25 )
I want to draw attention to the
id
field on this model. MongoDB uses _id
, but in Python, underscores at the start of attributes have special meaning. If you have an attribute on your model that starts with an underscore, pydantic—the data validation framework used by FastAPI—will assume that it is a private variable, meaning you will not be able to assign it a value! To get around this, we name the field id
but give it an alias of _id
. You also need to set populate_by_name
to True
in the model's model_config
We set this
id
value automatically to None
, so you do not need to supply it when creating a new student.1 class UpdateStudentModel(BaseModel): 2 """ 3 A set of optional updates to be made to a document in the database. 4 """ 5 6 name: Optional[str] = None 7 email: Optional[EmailStr] = None 8 course: Optional[str] = None 9 gpa: Optional[float] = None 10 model_config = ConfigDict( 11 arbitrary_types_allowed=True, 12 json_encoders={ObjectId: str}, 13 json_schema_extra={ 14 "example": { 15 "name": "Jane Doe", 16 "email": "jdoe@example.com", 17 "course": "Experiments, Science, and Fashion in Nanophotonics", 18 "gpa": 3.0, 19 } 20 }, 21 )
The
UpdateStudentModel
has two key differences from the StudentModel
:- It does not have an
id
attribute as this cannot be modified. - All fields are optional, so you only need to supply the fields you wish to update.
Finally,
StudentCollection
is defined to encapsulate a list of StudentModel
instances. In theory, the endpoint could return a top-level list of StudentModels, but there are some vulnerabilities associated with returning JSON responses with top-level lists.1 class StudentCollection(BaseModel): 2 """ 3 A container holding a list of `StudentModel` instances. 4 5 This exists because providing a top-level array in a JSON response can be a [vulnerability](https://haacked.com/archive/2009/06/25/json-hijacking.aspx/) 6 """ 7 8 students: List[StudentModel]
Our application has five routes:
- POST /students/ - creates a new student.
- GET /students/ - view a list of all students.
- GET /students/{id} - view a single student.
- PUT /students/{id} - update a student.
- DELETE /students/{id} - delete a student.
1 2 3 4 5 6 7 8 async def create_student(student: StudentModel = Body(...)): 9 """ 10 Insert a new student record. 11 12 A unique `id` will be created and provided in the response. 13 """ 14 new_student = await student_collection.insert_one( 15 student.model_dump(by_alias=True, exclude=["id"]) 16 ) 17 created_student = await student_collection.find_one( 18 {"_id": new_student.inserted_id} 19 ) 20 return created_student
The
create_student
route receives the new student data as a JSON string in a POST
request. We have to decode this JSON request body into a Python dictionary before passing it to our MongoDB client.The
insert_one
method response includes the _id
of the newly created student (provided as id
because this endpoint specifies response_model_by_alias=False
in the post
decorator call. After we insert the student into our collection, we use the inserted_id
to find the correct document and return this in our JSONResponse
.FastAPI returns an HTTP
200
status code by default; but in this instance, a 201
created is more appropriate.The application has two read routes: one for viewing all students and the other for viewing an individual student.
1 2 3 4 5 6 7 async def list_students(): 8 """ 9 List all of the student data in the database. 10 11 The response is unpaginated and limited to 1000 results. 12 """ 13 return StudentCollection(students=await student_collection.find().to_list(1000))
Motor's
to_list
method requires a max document count argument. For this example, I have hardcoded it to 1000
; but in a real application, you would use the skip and limit parameters in find
to paginate your results.1 2 3 4 5 6 7 async def show_student(id: str): 8 """ 9 Get the record for a specific student, looked up by `id`. 10 """ 11 if ( 12 student := await student_collection.find_one({"_id": ObjectId(id)}) 13 ) is not None: 14 return student 15 16 raise HTTPException(status_code=404, detail=f"Student {id} not found")
The student detail route has a path parameter of
id
, which FastAPI passes as an argument to the show_student
function. We use the id
to attempt to find the corresponding student in the database. The conditional in this section is using an assignment expression, an addition to Python 3.8 and often referred to by the cute sobriquet "walrus operator."If a document with the specified
_id
does not exist, we raise an HTTPException
with a status of 404
.1 2 3 4 5 6 7 async def update_student(id: str, student: UpdateStudentModel = Body(...)): 8 """ 9 Update individual fields of an existing student record. 10 11 Only the provided fields will be updated. 12 Any missing or `null` fields will be ignored. 13 """ 14 student = { 15 k: v for k, v in student.model_dump(by_alias=True).items() if v is not None 16 } 17 18 if len(student) >= 1: 19 update_result = await student_collection.find_one_and_update( 20 {"_id": ObjectId(id)}, 21 {"$set": student}, 22 return_document=ReturnDocument.AFTER, 23 ) 24 if update_result is not None: 25 return update_result 26 else: 27 raise HTTPException(status_code=404, detail=f"Student {id} not found") 28 29 # The update is empty, but we should still return the matching document: 30 if (existing_student := await student_collection.find_one({"_id": id})) is not None: 31 return existing_student 32 33 raise HTTPException(status_code=404, detail=f"Student {id} not found")
The
update_student
route is like a combination of the create_student
and the show_student
routes. It receives the id
of the document to update as well as the new data in the JSON body. We don't want to update any fields with empty values; so, first of all, we iterate over all the items in the received dictionary and only add the items that have a value to our new document.If, after we remove the empty values, there are no fields left to update, we instead look for an existing record that matches the
id
and return that unaltered. However, if there are values to update, we use find_one_and_update to $set the new values, and then return the updated document.If we get to the end of the function and we have not been able to find a matching document to update or return, then we raise a
404
error again.1 2 async def delete_student(id: str): 3 """ 4 Remove a single student record from the database. 5 """ 6 delete_result = await student_collection.delete_one({"_id": ObjectId(id)}) 7 8 if delete_result.deleted_count == 1: 9 return Response(status_code=status.HTTP_204_NO_CONTENT) 10 11 raise HTTPException(status_code=404, detail=f"Student {id} not found")
Our final route is
delete_student
. Again, because this is acting upon a single document, we have to supply an id
in the URL. If we find a matching document and successfully delete it, then we return an HTTP status of 204
or "No Content." In this case, we do not return a document as we've already deleted it! However, if we cannot find a student with the specified id
, then instead we return a 404
.If you're excited to build something more production-ready with FastAPI, React & MongoDB, head over to the Github repository for our new FastAPI app generator and start transforming your web development experience.
I hope you have found this introduction to FastAPI with MongoDB useful. If you would like to learn more, check out my post introducing the FARM stack (FastAPI, React and MongoDB) as well as the FastAPI documentation and this awesome list.
If you have questions, please head to our developer community website where MongoDB engineers and the MongoDB community will help you build your next big idea with MongoDB.