Go Driver Quick Start
On this page
This guide shows you how to create an application that uses the Go driver to connect to a MongoDB Atlas cluster. If you prefer to connect to MongoDB using a different driver or programming language, see our list of official MongoDB drivers.
The Go driver lets you connect to and communicate with MongoDB clusters from a Go application.
MongoDB Atlas is a fully-managed cloud database service that hosts your data on MongoDB clusters. In this guide, we show you how to get started with your own free (no credit card required) cluster.
Follow the steps below to connect your Go application with a MongoDB Atlas cluster.
Set Up Your Project
Use Go Mod to Initialize a Project
Create a new directory and initialize your project by using go mod
.
mkdir go-quickstart cd go-quickstart go mod init go-quickstart
Add MongoDB as a Dependency
Use go get
to add the Go driver as a dependency.
go get go.mongodb.org/mongo-driver/v2/mongo
Add Other Dependencies
Use go get
to add any additional dependencies. This Quick Start
uses the godotenv
package to read a MongoDB connection string
from an environment variable to avoid embedding credentials within
source code.
go get github.com/joho/godotenv
Create a MongoDB Cluster
Set up a Free Tier Cluster in Atlas
After setting up your Go project dependencies, create a MongoDB cluster where you can store and manage your data. Complete the Get Started with Atlas guide to set up a new Atlas account, free tier MongoDB cluster, load datasets, and interact with the data.
After completing the steps in the Atlas guide, you should have a new MongoDB cluster deployed in Atlas, a new database user, and sample datasets loaded into your cluster.
Connect to your Cluster
In this step, you create and run an application that uses the Go driver to connect to your MongoDB cluster and run a query on the sample data.
You pass instructions to the driver on where and how to connect to your MongoDB cluster in a string called the connection string. This string includes information on the hostname or IP address and port of your cluster, authentication mechanism, user credentials when applicable, and other connection options.
To retrieve your connection string for the cluster and user you created in the previous step, log into your Atlas account and navigate to the Database section and click the Connect button for the cluster that you want to connect to as shown below.

Proceed to the Connect Your Application step and select the Go driver. Then, click the Copy button to copy the connection string to your clipboard as shown below.
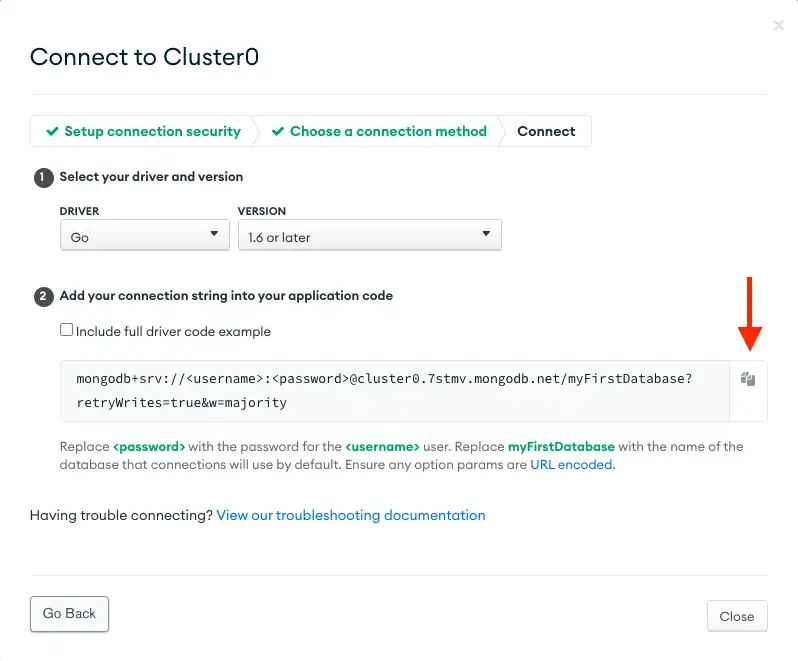
Save your Atlas connection string in a safe location that you can access for the next step.
To learn more about connecting to the Go driver through Atlas, see the Atlas driver connection guide and select Go from the Select your language dropdown.
Query Your MongoDB Cluster from Your Application
Next, create a file to contain your application called main.go
in the base directory of your project. Use the following sample
code to run a query on your sample dataset in MongoDB Atlas.
Specify your MongoDB Atlas connection string as the value of the
uri
variable, or create an environment variable called
MONGODB_URI
and set your Atlas connection string as its value.
export MONGODB_URI='<your atlas connection string>'
Note
Make sure to replace the "<password>" section of the connection string with the password you created for your user that has atlasAdmin permissions.
package main import ( "context" "encoding/json" "fmt" "log" "os" "github.com/joho/godotenv" "go.mongodb.org/mongo-driver/v2/bson" "go.mongodb.org/mongo-driver/v2/mongo" "go.mongodb.org/mongo-driver/v2/mongo/options" ) func main() { if err := godotenv.Load(); err != nil { log.Println("No .env file found") } uri := os.Getenv("MONGODB_URI") docs := "www.mongodb.com/docs/drivers/go/current/" if uri == "" { log.Fatal("Set your 'MONGODB_URI' environment variable. " + "See: " + docs + "usage-examples/#environment-variable") } client, err := mongo.Connect(options.Client(). ApplyURI(uri)) if err != nil { panic(err) } defer func() { if err := client.Disconnect(context.TODO()); err != nil { panic(err) } }() coll := client.Database("sample_mflix").Collection("movies") title := "Back to the Future" var result bson.M err = coll.FindOne(context.TODO(), bson.D{{"title", title}}). Decode(&result) if err == mongo.ErrNoDocuments { fmt.Printf("No document was found with the title %s\n", title) return } if err != nil { panic(err) } jsonData, err := json.MarshalIndent(result, "", " ") if err != nil { panic(err) } fmt.Printf("%s\n", jsonData) }
Run the sample code with the following command from your command line:
go run main.go
When you run main.go
, it should output the details of the movie from
the sample dataset which looks something like the following:
{ "_id": "573a1398f29313caabce9682", ... "title": "Back to the Future", ... }
If you receive no output or an error, check whether you properly set up your environment variable and whether you loaded the sample dataset in your MongoDB Atlas cluster.
Tip
If your output is empty, ensure you have loaded the sample datasets into your cluster.
After completing this step, you should have a working application that uses the Go driver to connect to your MongoDB cluster, run a query on the sample data, and print out the result.
Next steps
Learn how to read and modify data using the Go driver in our Fundamentals CRUD guide or how to perform common operations from our Usage Examples.