Modify Documents
On this page
Overview
In this guide, you can learn how to modify documents in MongoDB by using update and replace operations.
Update operations change the fields that you specify while leaving other
fields and values unchanged. Replace operations remove all existing
fields of a document except for the _id
field and substitute the
removed fields with new fields and values.
This guide includes the following sections:
Update Documents describes how to use the driver to execute update operations
Replace a Document describes how to use the driver to execute replace operations
Modify Update and Replace Behavior describes how to modify the default behavior of the methods described in this guide
Additional Information provides links to resources and API documentation for types and methods mentioned in this guide
Update Document Pattern
In MongoDB, all methods to change documents follow the same pattern:
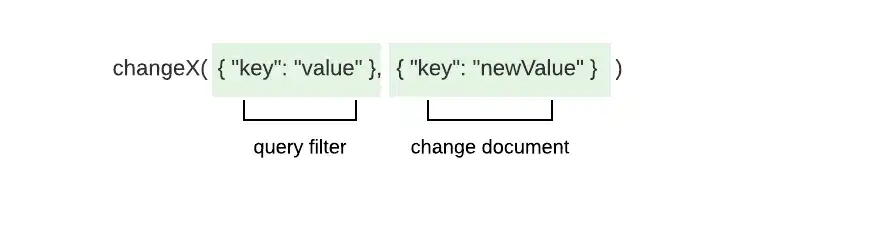
Note
changeX()
is a placeholder and not a real method.
These methods take the following parameters:
A query filter to match one or more documents to change
An update document that specifies the field and value changes
The Rust driver provides the following methods to change documents:
update_one()
update_many()
replace_one()
You can retrieve and modify data in one action by using compound operations. To learn more, see the guide on Compound Operations.
The _id Field
Each document in a MongoDB collection has a unique and immutable _id
field. If you attempt to change the _id
field through an update or
replace operation, the driver raises a WriteError
and performs no
updates.
Update Documents
You can perform update operations with the following methods:
update_one()
, which updates the first document that matches the search criteriaupdate_many()
, which updates all documents that match the search criteria
You can also chain option builder methods to these update operation methods. To learn about modifying the behavior of the update methods, see the Modify Update and Replace Behavior section of this guide.
Parameters
Each method takes a query filter and an update document that includes at least one update operator. The update operator specifies the type of update to perform and includes the fields and values that describe the change. Update documents use the following format:
doc! { "<update operator>": doc! { "<field>": <value> } }
To specify multiple updates in one update document, use the following format:
doc! { "<update operator>": doc!{"<field>": <value>}, "<update operator>": doc!{"<field>": <value>}, ... }
See the MongoDB server manual for a complete list of update operators and descriptions.
Note
Aggregation Pipelines in Update Operations
If you are using MongoDB Server version 4.2 or later, you can use aggregation pipelines in update operations. To learn more about the aggregation stages MongoDB supports in aggregation pipelines, see our tutorial on performing updates with aggregation pipelines.
Return Value
The update_one()
and update_many()
methods return an
UpdateResult
type if the operation is successful. The
UpdateResult
type contains the following properties that describe
the operation:
Property | Description |
---|---|
matched_count | The number of documents matched by the filter |
modified_count | The number of documents modified by the operation |
upserted_id | The _id of the upserted document, or empty if there is none |
If multiple documents match the query filter you pass to UpdateOne()
,
the method selects and updates the first matched document. If no
documents match the query filter, the update operation makes no
changes.
Update Example
The following documents describe employees of a company:
{ "_id": ObjectId('4337'), "name": "Shelley Olson", "department": "Marketing", "role": "Director", "bonus": 3000 }, { "_id": ObjectId('4902'), "name": "Remi Ibrahim", "department": "Marketing", "role": "Consultant", "bonus": 1800 }
This example performs an update operation with the update_many()
method.
The update_many()
method takes the following parameters:
A query filter to match documents where the value of the
department
field is"Marketing"
An update document that contains the following updates:
A
$set
operator to change the value ofdepartment
to"Business Operations"
androle
to"Analytics Specialist"
An
$inc
operator to increase the value ofbonus
by500
let update_doc = doc! { "$set": doc! { "department": "Business Operations", "role": "Analytics Specialist" }, "$inc": doc! { "bonus": 500 } }; let res = my_coll .update_many(doc! { "department": "Marketing" }, update_doc) .await?; println!("Modified documents: {}", res.modified_count);
Modified documents: 2
The following documents reflect the changes resulting from the preceding update operation:
{ "_id": ObjectId('4337'), "name": "Shelley Olson", "department": "Business Operations", "role": "Analytics Specialist", "bonus": 3500 }, { "_id": ObjectId('4902'), "name": "Remi Ibrahim", "department": "Business Operations", "role": "Analytics Specialist", "bonus": 2300 }
Update by ObjectId Example
The following document describes an employee of a company:
{ "_id": ObjectId('4274'), "name": "Jill Millerton", "department": "Marketing", "role": "Consultant" }
This example queries for the preceding document by specifying a query filter to match the
document's unique _id
value. Then, the code performs an update operation with the
update_one()
method. The update_one()
method takes the following parameters:
Query filter that matches a document in which the value of the
_id
field isObjectId('4274')
Update document that creates instructions to set the value of
name
to"Jill Gillison"
let id = ObjectId::from_str("4274").expect("Could not convert to ObjectId"); let filter_doc = doc! { "_id": id }; let update_doc = doc! { "$set": doc! { "name": "Jill Gillison" } }; let res = my_coll .update_one(filter_doc, update_doc) .await?; println!("Modified documents: {}", res.modified_count);
Modified documents: 1
The following document reflects the changes resulting from the preceding update operation:
{ "_id": ObjectId('4274'), "name": "Jill Gillison", "department": "Marketing", "role": "Consultant" }
Tip
To learn more about the _id
field, see the _id Field
section of this page or the ObjectId()
method documentation in the Server manual.
Replace a Document
You can perform a replace operation with the replace_one()
method.
This method replaces all existing fields of a document except for the _id
field with new fields and values that you specify.
You can also chain option builder methods to a replace operation method. To learn
about modifying the behavior of the replace_one()
method, see the Modify
Update and Replace Behavior section of this guide.
Parameters
The replace_one()
method takes a query filter and a replacement
document, which contains the fields and values that will replace an
existing document. Replacement documents use the following format:
doc! { "<field>": <value>, "<field>": <value>, ... }
Return Values
The replace_one
method returns an
UpdateResult
type if the operation is successful. The
UpdateResult
type contains the following properties that describe
the operation:
Property | Description |
---|---|
matched_count | The number of documents matched by the filter |
modified_count | The number of documents modified by the operation |
upserted_id | The _id of the upserted document, or empty if there is none |
If multiple documents match the query filter you pass to replace_one()
,
the method selects and replaces the first matched document. If no
documents match the query filter, the replace operation makes no
changes.
Replace Example
The following document describes an employee of a company:
{ "_id": ObjectId('4501'), "name": "Matt DeGuy", "role": "Consultant", "team_members": [ "Jill Gillison", "Susan Lee" ] }
This example uses the replace_one()
method to replace
the preceding document with one that has the following fields:
A
name
value of"Susan Lee"
A
role
value of"Lead Consultant"
A
team_members
value of[ "Jill Gillison" ]
let replace_doc = doc! { "name": "Susan Lee", "role": "Lead Consultant", "team_members": vec! [ "Jill Gillison" ] }; let res = my_coll .replace_one(doc! { "name": "Matt DeGuy" }, replace_doc) .await?; println!( "Matched documents: {}\nModified documents: {}", res.matched_count, res.modified_count );
Matched documents: 1 Modified documents: 1
The replaced document contains the contents of the replacement document
and the immutable _id
field:
{ "_id": ObjectId('4501'), "name": "Susan Lee", "role": "Lead Consultant", "team_members": [ "Jill Gillison" ] }
Modify Update and Replace Behavior
You can modify the behavior of the update_one()
, update_many
,
and replace_one()
methods by calling options methods that set
UpdateOptions
struct fields.
Note
Setting Options
You can set UpdateOptions
fields by chaining option builder methods directly
to the update or replace method call. If you're using an earlier version of the driver,
you must construct an UpdateOptions
instance by chaining option builder methods
to the builder()
method. Then, pass your options instance as a parameter to
update_one()
, update_many
, or replace_one()
.
The following table describes the options available in UpdateOptions
:
Option | Description |
---|---|
array_filters | The set of filters specifying the array elements to which the
update applies. Type: Vec<Document> |
bypass_document_validation | If true , allows the driver to perform a write that violates
document-level validation. To learn more about validation, see
the guide on Schema Validation.Type: bool Default: false |
upsert | If true, the operation inserts a document if no documents match
the query filter. Type: bool |
collation | The collation to use when sorting results. To learn more about collations,
see the Collations guide. Type: Collation Default: None |
hint | The index to use for the operation. This option is available
only when connecting to MongoDB Server versions 4.2 and later. Type: Hint Default: None |
write_concern | The write concern for the operation. If you don't set this
option, the operation inherits the write concern set for
the collection. To learn more about write concerns, see
Write Concern in the
Server manual. Type: WriteConcern |
let_vars | A map of parameters and values. These parameters can be accessed
as variables in aggregation expressions. This option is available
only when connecting to MongoDB Server versions 5.0 and later. Type: Document |
comment | An arbitrary Bson value tied to the operation to trace
it through the database profiler, currentOp , and
logs. This option is available only when connecting to
MongoDB Server versions 4.4 and later.Type: Bson Default: None |
The following code shows how to set the upsert
field by chaining
the upsert()
method to the update_one()
method:
let res = my_coll .update_one(filter_doc, update_doc) .upsert(true) .await?;
Additional Information
For more information about the concepts in this guide, see the following documentation:
Specify a Query guide
Compound Operations guide
For runnable examples of the update and replace operations, see the following usage examples:
To learn more about the update operators, see Update Operators in the Server manual.
API Documentation
To learn more about the methods and types mentioned in this guide, see the following API documentation: