Analyze LINQ Expressions
On this page
Overview
LINQ is a query syntax included in the C# language. The .NET/C# driver can translate a subset of LINQ expressions into MongoDB aggregation pipelines.
To learn more about LINQ, see the following resources:
To learn more about aggregation pipelines, see Aggregation in the MongoDB manual.
Note
Runtime Differences
Although the MongoDB Query API translations generated by the C# Analyzer have the same query shape as your runtime .NET/C# driver queries, there may be slight differences due to the following factors:
Your Serialization Settings
Your LINQ Settings
The difference between the translations the C# Analyzer generates and your queries at runtime should not impact your ability to analyze and debug your code.
To learn more about serialization and LINQ settings, see the FAQ page.
Use the C# Analyzer to learn the following about your LINQ expressions:
How your LINQ expressions translate into the MongoDB Query API
If any of your LINQ expressions are not supported
Translate into the MongoDB Query API
Click the following tabs to see an example of a LINQ expression and its corresponding MongoDB Query API translation:
var books = queryableCollection .Where(b => b.Genre == genre && b.Price >= minPrice && b.Title.Contains(titleSearchTerm)) .OrderBy(b => b.Price) .ToList();
[{ "$match" : { "Genre" : genre, "Price" : { "$gte" : minPrice }, "Title" : /titleSearchTerm/s } }, { "$sort" : { "Price" : 1 } }]
Note
Variable Names
The MongoDB Query API translations generated by the C# Analyzer contain variable names from your .NET/C# driver code. The .NET/C# driver replaces these variable names with their corresponding values when your application communicates with MongoDB.
Analyze LINQ in Visual Studio
To analyze your LINQ expressions in Visual Studio, perform the following actions:
Install the C# Analyzer as described in the Install guide.
Write a LINQ expression with the .NET/C# driver.
Move your mouse over the ... annotation beneath the first method of your LINQ expression to display an information message that contains the MongoDB Query API translation.
Simple LINQ Expressions
The C# Analyzer analyzes LINQ expressions in the following syntax types:
Method syntax
Query syntax
Click on the following corresponding tab to see a LINQ expression written in method syntax with or without an information message displayed:
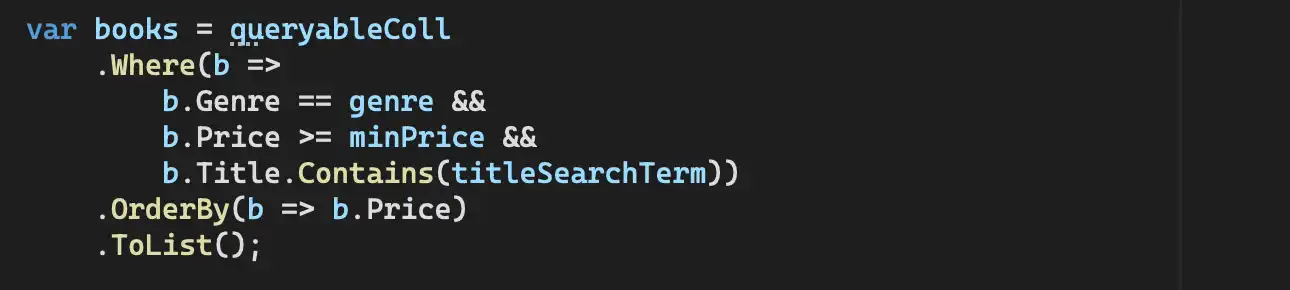
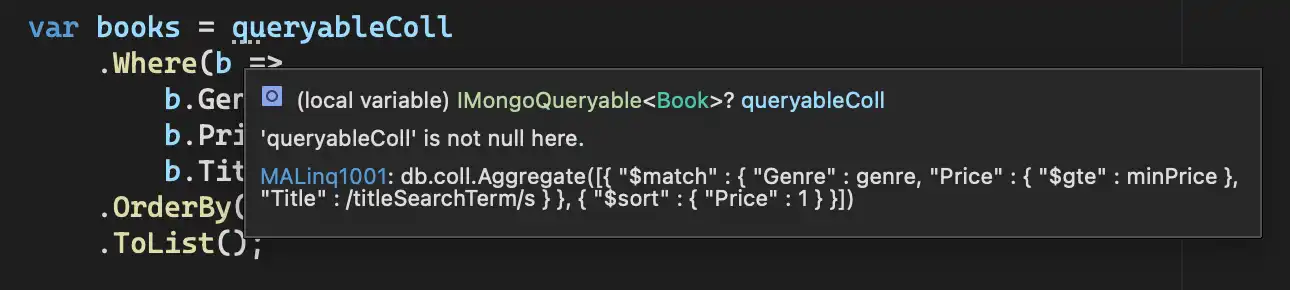
Click on the following corresponding tab to see a LINQ expression written in query syntax with or without an information message displayed:


Unsupported LINQ Expressions
If your LINQ expression is not supported, the C# Analyzer outputs a
NotSupportedLinqExpression
warning.
Click the following tabs to see a code snippet containing an unsupported LINQ expression and the corresponding warning message displayed by the C# Analyzer:
The following code snippet contains the unsupported GetHashCode
LINQ expression:
var result = queryableColl.Where(b => b.GetHashCode() == 167);
The following screenshot shows the annotation displayed by the C# Analyzer underneath the preceding code snippet in Visual Studio:

The following is the warning generated by the C# Analyzer:
Expression not supported: b.GetHashCode().
The following screenshot shows the warning displayed in Visual Studio:
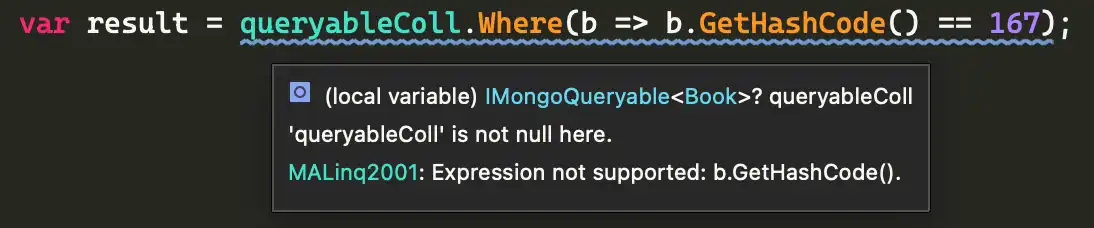
Tip
Error List Panel
If you are using Visual Studio for Windows, you can view the output from the {+product+-short} in the Error List window.
To learn more, see Error List Window from Microsoft.
To view more examples of unsupported LINQ expressions, see the MongoDB C# Analyzer Github repository.
Analyze LINQ3
To analyze a LINQ3 expression, you must configure the C# Analyzer to use the LINQ3 provider. To learn how to configure your LINQ provider, see the configuration guide.
Important
Expressions Supported Only by LINQ3
If your .NET/C# driver version supports LINQ3 but you configure your C# Analyzer to use the default LINQ provider (LINQ2), the C# Analyzer informs you if your LINQ expression is supported by LINQ3 but not LINQ2.
Click the tabs to see a LINQ expression supported by LINQ3 but not LINQ2 and the corresponding warning output by the C# Analyzer:
var result = queryableColl.Where(b => b.Title.Substring(0) == "Pane");
Supported in LINQ3 only: db.coll.Aggregate([{ "$match" : { "$expr" : { "$eq" : [{ "$substrCP" : ["$Title", 0, { "$subtract" : [{ "$strLenCP" : "$Title" }, 0] }] }, "Pane"] } } }])
To view examples of expressions the .NET/C# driver only supports with the LINQ3 provider, see the MongoDB C# Analyzer Github repository.