マルチユーザー アプリケーション - Java SDK
Realm SDK を使用すると、複数のユーザーが特定のデバイスで同時にアプリにログインできます。 Realm クライアント アプリケーションは、複数のユーザーが同時にログインしている場合でも、単一のアクティブ ユーザーのコンテキストで実行されます。 再度ログインする必要なく、認証されたユーザー間ですばやく切り替えることができます。
警告
ログインしたユーザーは、再認証を行わずに アクティブ ユーザー になる場合があります。 アプリによっては、セキュリティ上の脆弱性がある場合があります。 たとえば、共有デバイス上のユーザーは、認証情報を提供したり明示的な許可を必要とせずに、同僚のログイン アカウントに切り替えることができます。 アプリケーションでより厳格な認証が必要な場合は、ユーザー間の切り替えを避け、別のユーザーを認証する前にアクティブなユーザーを明示的にログアウトすることをおすすめします。
ユーザー アカウントの状態
ユーザーが特定のデバイスまたはブラウザで Realm SDK を介して初めてログインすると、SDK はユーザーの情報を保存し、デバイス上のユーザーの状態を追跡します。 ログアウトした場合でも、ユーザーをアクティブに削除しない限り、ユーザーのデータはデバイス上に残ります。
次の状態では、ある時点でデバイス上のユーザーを記述します。
認証済み:デバイスにログイン済みで、ログアウトしたことがない、またはセッションが取り消されているユーザー。
アクティブ: 特定のデバイスで現在アプリを使用している単一の認証済みユーザー。 SDK はこのユーザーを送信リクエストに関連付け、Atlas App Services はデータアクセス権限を評価し、このユーザーのコンテキストで関数を実行します。 詳細については、アクティブ ユーザーを参照してください。
非アクティブ: 現在アクティブなユーザーではない、すべての認証済みユーザー。 現在非アクティブなユーザーにいつでもアクティブなユーザーを切り替えることができます。
ログアウト:デバイスで認証されたが、ログアウトされたユーザーまたはセッションが取り消されたユーザー。
以下の図は、特定のイベントが発生したときに Realm クライアント アプリ内のユーザーが状態間でどのように移行するかを示しています。
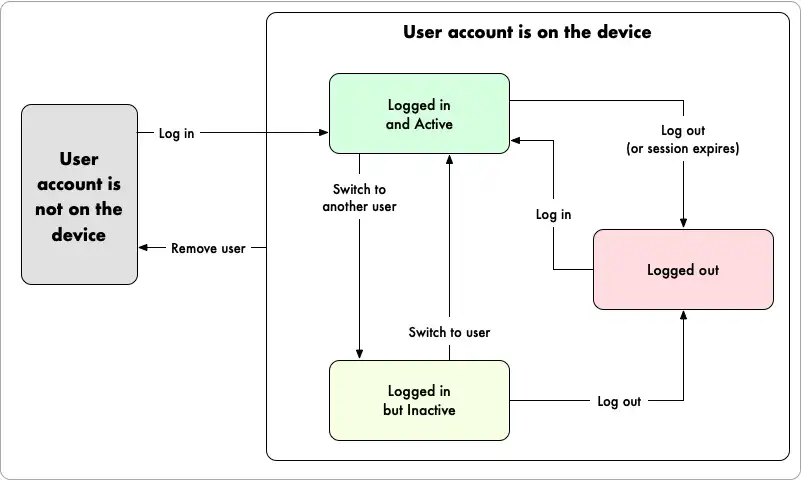
デバイスへの新しいユーザーの追加
Realm SDK は、ユーザーがそのデバイスに初めてログインするときに、そのデバイスにユーザーを自動的に追加します。 ユーザーはログインすると、すぐにアプリケーションのアクティブ ユーザー になります。
String appID = YOUR_APP_ID; // replace this with your App ID App app = new App(new AppConfiguration.Builder(appID).build()); // Log in as Joe Credentials joeCredentials = Credentials.emailPassword(firstUserEmail, firstUserPassword); app.loginAsync(joeCredentials, it -> { if (it.isSuccess()) { // The active user is now Joe User joe = it.get(); assert joe == app.currentUser(); } else { Log.e("EXAMPLE", "Failed to log in: " + it.getError().getErrorMessage()); } }); // Log in as Emma Credentials emmaCredentials = Credentials.emailPassword(secondUserEmail, secondUserPassword); app.loginAsync(emmaCredentials, it -> { if (it.isSuccess()) { // The active user is now Emma User emma = it.get(); assert emma == app.currentUser(); } else { Log.e("EXAMPLE", "Failed to log in: " + it.getError().getErrorMessage()); } });
val appID: String = YOUR_APP_ID // replace this with your App ID val app = App(AppConfiguration.Builder(appID).build()) // Log in as Joe val joeCredentials = Credentials.emailPassword(firstUserEmail, firstUserPassword) app.loginAsync(joeCredentials) { if (it.isSuccess) { // The active user is now Joe val joe = it.get() assert(joe === app.currentUser()) } else { Log.e("EXAMPLE", "Failed to log in: ${it.error.errorMessage}") } } // Log in as Emma val emmaCredentials = Credentials.emailPassword(secondUserEmail, secondUserPassword) app.loginAsync(emmaCredentials) { if (it.isSuccess) { // The active user is now Emma val emma = it.get() assert(emma === app.currentUser()) } else { Log.e("EXAMPLE", "Failed to log in: ${it.error.errorMessage}") } }
デバイス上のすべてのユーザーを一覧表示
デバイスに保存されているすべてのユーザー アカウントのリストにアクセスできます。 このリストには、現在認証されているかどうかに関係なく、特定のデバイス上のクライアントアプリにログインしたすべてのユーザーが含まれます。
Map<String, User> users = app.allUsers(); for (Map.Entry<String, User> user : users.entrySet()) { Log.v("EXAMPLE", "User: " + user.getKey()); }
val users = app.allUsers() for ((key) in users) { Log.v("EXAMPLE", "User: $key") }
デバイスからユーザーを削除
デバイスからユーザーに関するすべての情報を削除するには、 user.remove()またはuser.removeAsync( ) を使用します。
app.loginAsync(credentials, it -> { if (it.isSuccess()) { User user = it.get(); user.removeAsync(result -> { if (result.isSuccess()) { Log.v("EXAMPLE", "Successfully removed user from device."); } else { Log.e("EXAMPLE", "Failed to remove user from device."); } }); } else { Log.e("EXAMPLE", "Failed to log in: " + it.getError().getErrorMessage()); } });
app.loginAsync(credentials) { if (it.isSuccess) { val user = it.get() user.removeAsync { result: App.Result<User?> -> if (result.isSuccess) { Log.v("EXAMPLE", "Successfully removed user from device.") } else { Log.e("EXAMPLE", "Failed to remove user from device.") } } } else { Log.e("EXAMPLE", "Failed to log in: ${it.error.errorMessage}") } }
アクティブなユーザーの変更
アプリのアクティブ ユーザーを別のログイン ユーザーにいつでもすばやく切り替えることができます。
// Joe is already logged in and is the currently active user User joe = app.currentUser(); // Log in as Emma Credentials emmaCredentials = Credentials.emailPassword(secondUserEmail, secondUserPassword); app.loginAsync(emmaCredentials, result -> { if (result.isSuccess()) { // The active user is now Emma User emma = result.get(); assert emma == app.currentUser(); // Switch active user back to Joe app.switchUser(joe); assert joe == app.currentUser(); } else { Log.e("EXAMPLE", "Failed to log in: " + result.getError().getErrorMessage()); } });
// Joe is already logged in and is the currently active user val joe = app.currentUser() // Log in as Emma val emmaCredentials = Credentials.emailPassword( secondUserEmail, secondUserPassword ) app.loginAsync(emmaCredentials) { result -> if (result.isSuccess) { // The active user is now Emma val emma = result.get() assert(emma === app.currentUser()) // Switch active user back to Joe app.switchUser(joe) assert(joe === app.currentUser()) } else { Log.e("EXAMPLE", "Failed to log in: ${result.error.errorMessage}") } }