複数のユーザーとの連携 - Web SDK
Overview
Realm SDK を使用すると、複数のユーザーが特定のブラウザで同時にアプリにログインできます。 Realm クライアント アプリケーションは、複数のユーザーが同時にログインしている場合でも、単一のアクティブ ユーザーのコンテキストで実行されます。 再度ログインする必要なく、認証されたユーザー間ですばやく切り替えることができます。
重要
ログインしたユーザーは、再認証を行わずに アクティブ ユーザー になる場合があります。 アプリによっては、セキュリティ上の脆弱性がある場合があります。 たとえば、共有ブラウザを使用しているユーザーは、認証情報を提供したり明示的な許可を必要とせずに、同僚のログイン アカウントに切り替えることができます。 アプリケーションでより厳格な認証が必要な場合は、ユーザー間の切り替えを避け、別のユーザーを認証する前にアクティブなユーザーを明示的にログアウトすることをおすすめします。
ユーザー アカウントの状態
ユーザーが初めて特定のブラウザで Realm SDK 経由でログインすると、SDK はユーザーの情報を保存し、ユーザーの状態を追跡します。 ユーザーがログアウトした場合でも、ユーザーをアクティブに削除したり、ブラウザからデータを消去したりしない限り、ユーザーのデータはローカル ストレージに残ります。
次の状態では、いつでも追跡されているユーザーを説明します。
認証済み:ブラウザーにログインし、ログアウトしたり、セッションを取り消したりしていないユーザー。
アクティブ: 特定のブラウザでアプリを現在使用している単一の認証ユーザー。 SDK はこのユーザーを送信リクエストに関連付け、Atlas App Services はデータアクセス権限を評価し、このユーザーのコンテキストで関数を実行します。 詳細については、アクティブ ユーザーを参照してください。
非アクティブ: 現在アクティブなユーザーではない、すべての認証済みユーザー。 現在非アクティブなユーザーにいつでもアクティブなユーザーを切り替えることができます。
ログアウト:ブラウザで認証されたが、ログアウトされたユーザーまたはセッションが取り消されたユーザー。
以下の図は、特定のイベントが発生したときに Realm クライアント アプリ内のユーザーが状態間でどのように移行するかを示しています。
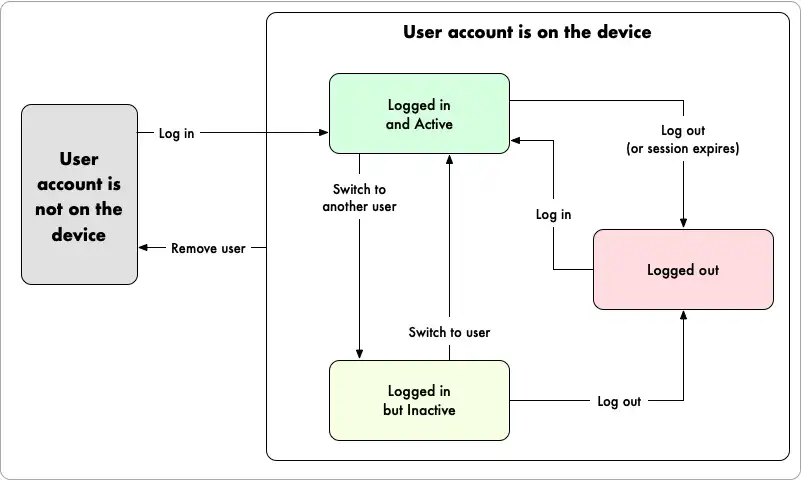
デバイスへの新しいユーザーの追加
Realm SDK は、ユーザーがそのブラウザに初めてログインするときに、ユーザー データをブラウザのローカル ストレージに自動的に保存します。 ユーザーはログインすると、すぐにアプリケーションのアクティブ ユーザー になります。
// Register Joe const joeEmail = "joe@example.com"; const joePassword = "passw0rd"; await app.emailPasswordAuth.registerUser({ email: joeEmail, password: joePassword, }); // Log in as Joe const joeCredentials = Realm.Credentials.emailPassword( joeEmail, joePassword ); const joe = await app.logIn(joeCredentials); // The active user is now Joe console.assert(joe.id === app.currentUser.id); // Register Emma const emmaEmail = "emma@example.com"; const emmaPassword = "passw0rd"; await app.emailPasswordAuth.registerUser({ email: emmaEmail, password: emmaPassword, }); // Log in as Emma const emmaCredentials = Realm.Credentials.emailPassword( emmaEmail, emmaPassword ); const emma = await app.logIn(emmaCredentials); // The active user is now Emma, but Joe is still logged in console.assert(emma.id === app.currentUser.id);
すべてのオンデバイス ユーザーを一覧表示
ブラウザに関連付けられているすべてのユーザー アカウントのリストにアクセスできます。 このリストには、現在認証されているかどうかに関係なく、クライアント アプリにログインしたすべてのユーザーが含まれます。
// Get an object with all Users, where the keys are the User IDs for (const userId in app.allUsers) { const user = app.allUsers[userId]; console.log( `User with id ${user.id} is ${ user.isLoggedIn ? "logged in" : "logged out" }` ); }
アクティブなユーザーの切り替え
アプリのアクティブ ユーザーを別のログイン ユーザーにいつでもすばやく切り替えることができます。
// Get some logged-in users const authenticatedUsers = Object.values(app.allUsers).filter( (user) => user.isLoggedIn ); const user1 = authenticatedUsers[0]; const user2 = authenticatedUsers[1]; // Switch to user1 app.switchUser(user1); // The active user is now user1 console.assert(app.currentUser.id === user1.id); // Switch to user2 app.switchUser(user2); // The active user is now user2 console.assert(app.currentUser.id === user2.id);
デバイスからユーザーを削除
ブラウザからユーザーに関するすべての情報を削除し、ユーザーを自動的にログアウトできます。
// Remove the current user from the device const user = app.currentUser; await app.removeUser(user); // The user is no longer the active user if (app.currentUser) { // The active user is now the logged in user (if there still is one) that was // most recently active console.assert(user.id !== app.currentUser.id); } // The user is no longer on the device console.assert( Object.values(app.allUsers).find(({ id }) => id === user.id) === undefined );