Update Documents
You can update documents in MongoDB using the following methods:
Your programming language's driver.
The MongoDB Atlas UI. To learn more, see Update a Document with MongoDB Atlas.
➤ Use the Select your language drop-down menu in the upper-right to set the language of the following examples.
This page uses the following mongo
methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses MongoDB Compass to update the documents.
The examples on this page use the inventory
collection.
Populate the inventory
collection with the following
documents:
This page uses the following MongoDB C# Driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following MongoDB Go Driver functions:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following Java Reactive Streams Driver methods:
com.mongodb.reactivestreams.client.MongoCollection.updateOne
com.mongodb.reactivestreams.client.MongoCollection.updateMany
com.mongodb.reactivestreams.client.MongoCollection.replaceOne
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following Java Synchronous Driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following Motor driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following MongoDB Node.js Driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following MongoDB Perl Driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following MongoDB PHP Library methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following PyMongo Python driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following MongoDB Ruby Driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
This page uses the following MongoDB Scala Driver methods:
The examples on this page use the
inventory
collection. Connect to a test database in your MongoDB
instance then create the inventory
collection:
db.inventory.insertMany( [ { item: "canvas", qty: 100, size: { h: 28, w: 35.5, uom: "cm" }, status: "A" }, { item: "journal", qty: 25, size: { h: 14, w: 21, uom: "cm" }, status: "A" }, { item: "mat", qty: 85, size: { h: 27.9, w: 35.5, uom: "cm" }, status: "A" }, { item: "mousepad", qty: 25, size: { h: 19, w: 22.85, uom: "cm" }, status: "P" }, { item: "notebook", qty: 50, size: { h: 8.5, w: 11, uom: "in" }, status: "P" }, { item: "paper", qty: 100, size: { h: 8.5, w: 11, uom: "in" }, status: "D" }, { item: "planner", qty: 75, size: { h: 22.85, w: 30, uom: "cm" }, status: "D" }, { item: "postcard", qty: 45, size: { h: 10, w: 15.25, uom: "cm" }, status: "A" }, { item: "sketchbook", qty: 80, size: { h: 14, w: 21, uom: "cm" }, status: "A" }, { item: "sketch pad", qty: 95, size: { h: 22.85, w: 30.5, uom: "cm" }, status: "A" } ] );
[ { "item": "canvas", "qty": 100, "size": { "h": 28, "w": 35.5, "uom": "cm" }, "status": "A" }, { "item": "journal", "qty": 25, "size": { "h": 14, "w": 21, "uom": "cm" }, "status": "A" }, { "item": "mat", "qty": 85, "size": { "h": 27.9, "w": 35.5, "uom": "cm" }, "status": "A" }, { "item": "mousepad", "qty": 25, "size": { "h": 19, "w": 22.85, "uom": "cm" }, "status": "P" }, { "item": "notebook", "qty": 50, "size": { "h": 8.5, "w": 11, "uom": "in" }, "status": "P" }, { "item": "paper", "qty": 100, "size": { "h": 8.5, "w": 11, "uom": "in" }, "status": "D" }, { "item": "planner", "qty": 75, "size": { "h": 22.85, "w": 30, "uom": "cm" }, "status": "D" }, { "item": "postcard", "qty": 45, "size": { "h": 10, "w": 15.25, "uom": "cm" }, "status": "A" }, { "item": "sketchbook", "qty": 80, "size": { "h": 14, "w": 21, "uom": "cm" }, "status": "A" }, { "item": "sketch pad", "qty": 95, "size": { "h": 22.85, "w": 30.5, "uom": "cm" }, "status": "A" } ]
For instructions on inserting documents using MongoDB Compass, see Insert Documents.
var documents = new[] { new BsonDocument { { "item", "canvas" }, { "qty", 100 }, { "size", new BsonDocument { { "h", 28 }, { "w", 35.5 }, { "uom", "cm" } } }, { "status", "A" } }, new BsonDocument { { "item", "journal" }, { "qty", 25 }, { "size", new BsonDocument { { "h", 14 }, { "w", 21 }, { "uom", "cm" } } }, { "status", "A" } }, new BsonDocument { { "item", "mat" }, { "qty", 85 }, { "size", new BsonDocument { { "h", 27.9 }, { "w", 35.5 }, { "uom", "cm" } } }, { "status", "A" } }, new BsonDocument { { "item", "mousepad" }, { "qty", 25 }, { "size", new BsonDocument { { "h", 19 }, { "w", 22.85 }, { "uom", "cm" } } }, { "status", "P" } }, new BsonDocument { { "item", "notebook" }, { "qty", 50 }, { "size", new BsonDocument { { "h", 8.5 }, { "w", 11 }, { "uom", "in" } } }, { "status", "P" } }, new BsonDocument { { "item", "paper" }, { "qty", 100 }, { "size", new BsonDocument { { "h", 8.5 }, { "w", 11 }, { "uom", "in" } } }, { "status", "D" } }, new BsonDocument { { "item", "planner" }, { "qty", 75 }, { "size", new BsonDocument { { "h", 22.85 }, { "w", 30 }, { "uom", "cm" } } }, { "status", "D" } }, new BsonDocument { { "item", "postcard" }, { "qty", 45 }, { "size", new BsonDocument { { "h", 10 }, { "w", 15.25 }, { "uom", "cm" } } }, { "status", "A" } }, new BsonDocument { { "item", "sketchbook" }, { "qty", 80 }, { "size", new BsonDocument { { "h", 14 }, { "w", 21 }, { "uom", "cm" } } }, { "status", "A" } }, new BsonDocument { { "item", "sketch pad" }, { "qty", 95 }, { "size", new BsonDocument { { "h", 22.85 }, { "w", 30.5 }, { "uom", "cm" } } }, { "status", "A" } }, }; collection.InsertMany(documents);
docs := []interface{}{ bson.D{ {"item", "canvas"}, {"qty", 100}, {"size", bson.D{ {"h", 28}, {"w", 35.5}, {"uom", "cm"}, }}, {"status", "A"}, }, bson.D{ {"item", "journal"}, {"qty", 25}, {"size", bson.D{ {"h", 14}, {"w", 21}, {"uom", "cm"}, }}, {"status", "A"}, }, bson.D{ {"item", "mat"}, {"qty", 85}, {"size", bson.D{ {"h", 27.9}, {"w", 35.5}, {"uom", "cm"}, }}, {"status", "A"}, }, bson.D{ {"item", "mousepad"}, {"qty", 25}, {"size", bson.D{ {"h", 19}, {"w", 22.85}, {"uom", "in"}, }}, {"status", "P"}, }, bson.D{ {"item", "notebook"}, {"qty", 50}, {"size", bson.D{ {"h", 8.5}, {"w", 11}, {"uom", "in"}, }}, {"status", "P"}, }, bson.D{ {"item", "paper"}, {"qty", 100}, {"size", bson.D{ {"h", 8.5}, {"w", 11}, {"uom", "in"}, }}, {"status", "D"}, }, bson.D{ {"item", "planner"}, {"qty", 75}, {"size", bson.D{ {"h", 22.85}, {"w", 30}, {"uom", "cm"}, }}, {"status", "D"}, }, bson.D{ {"item", "postcard"}, {"qty", 45}, {"size", bson.D{ {"h", 10}, {"w", 15.25}, {"uom", "cm"}, }}, {"status", "A"}, }, bson.D{ {"item", "sketchbook"}, {"qty", 80}, {"size", bson.D{ {"h", 14}, {"w", 21}, {"uom", "cm"}, }}, {"status", "A"}, }, bson.D{ {"item", "sketch pad"}, {"qty", 95}, {"size", bson.D{ {"h", 22.85}, {"w", 30.5}, {"uom", "cm"}, }}, {"status", "A"}, }, } result, err := coll.InsertMany(context.TODO(), docs)
Publisher<Success> insertManyPublisher = collection.insertMany(asList( Document.parse("{ item: 'canvas', qty: 100, size: { h: 28, w: 35.5, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'journal', qty: 25, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'mat', qty: 85, size: { h: 27.9, w: 35.5, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'mousepad', qty: 25, size: { h: 19, w: 22.85, uom: 'cm' }, status: 'P' }"), Document.parse("{ item: 'notebook', qty: 50, size: { h: 8.5, w: 11, uom: 'in' }, status: 'P' }"), Document.parse("{ item: 'paper', qty: 100, size: { h: 8.5, w: 11, uom: 'in' }, status: 'D' }"), Document.parse("{ item: 'planner', qty: 75, size: { h: 22.85, w: 30, uom: 'cm' }, status: 'D' }"), Document.parse("{ item: 'postcard', qty: 45, size: { h: 10, w: 15.25, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'sketchbook', qty: 80, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'sketch pad', qty: 95, size: { h: 22.85, w: 30.5, uom: 'cm' }, status: 'A' }") ));
collection.insertMany(asList( Document.parse("{ item: 'canvas', qty: 100, size: { h: 28, w: 35.5, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'journal', qty: 25, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'mat', qty: 85, size: { h: 27.9, w: 35.5, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'mousepad', qty: 25, size: { h: 19, w: 22.85, uom: 'cm' }, status: 'P' }"), Document.parse("{ item: 'notebook', qty: 50, size: { h: 8.5, w: 11, uom: 'in' }, status: 'P' }"), Document.parse("{ item: 'paper', qty: 100, size: { h: 8.5, w: 11, uom: 'in' }, status: 'D' }"), Document.parse("{ item: 'planner', qty: 75, size: { h: 22.85, w: 30, uom: 'cm' }, status: 'D' }"), Document.parse("{ item: 'postcard', qty: 45, size: { h: 10, w: 15.25, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'sketchbook', qty: 80, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }"), Document.parse("{ item: 'sketch pad', qty: 95, size: { h: 22.85, w: 30.5, uom: 'cm' }, status: 'A' }") ));
await db.inventory.insert_many( [ { "item": "canvas", "qty": 100, "size": {"h": 28, "w": 35.5, "uom": "cm"}, "status": "A", }, { "item": "journal", "qty": 25, "size": {"h": 14, "w": 21, "uom": "cm"}, "status": "A", }, { "item": "mat", "qty": 85, "size": {"h": 27.9, "w": 35.5, "uom": "cm"}, "status": "A", }, { "item": "mousepad", "qty": 25, "size": {"h": 19, "w": 22.85, "uom": "cm"}, "status": "P", }, { "item": "notebook", "qty": 50, "size": {"h": 8.5, "w": 11, "uom": "in"}, "status": "P", }, { "item": "paper", "qty": 100, "size": {"h": 8.5, "w": 11, "uom": "in"}, "status": "D", }, { "item": "planner", "qty": 75, "size": {"h": 22.85, "w": 30, "uom": "cm"}, "status": "D", }, { "item": "postcard", "qty": 45, "size": {"h": 10, "w": 15.25, "uom": "cm"}, "status": "A", }, { "item": "sketchbook", "qty": 80, "size": {"h": 14, "w": 21, "uom": "cm"}, "status": "A", }, { "item": "sketch pad", "qty": 95, "size": {"h": 22.85, "w": 30.5, "uom": "cm"}, "status": "A", }, ] )
await db.collection('inventory').insertMany([ { item: 'canvas', qty: 100, size: { h: 28, w: 35.5, uom: 'cm' }, status: 'A' }, { item: 'journal', qty: 25, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }, { item: 'mat', qty: 85, size: { h: 27.9, w: 35.5, uom: 'cm' }, status: 'A' }, { item: 'mousepad', qty: 25, size: { h: 19, w: 22.85, uom: 'cm' }, status: 'P' }, { item: 'notebook', qty: 50, size: { h: 8.5, w: 11, uom: 'in' }, status: 'P' }, { item: 'paper', qty: 100, size: { h: 8.5, w: 11, uom: 'in' }, status: 'D' }, { item: 'planner', qty: 75, size: { h: 22.85, w: 30, uom: 'cm' }, status: 'D' }, { item: 'postcard', qty: 45, size: { h: 10, w: 15.25, uom: 'cm' }, status: 'A' }, { item: 'sketchbook', qty: 80, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }, { item: 'sketch pad', qty: 95, size: { h: 22.85, w: 30.5, uom: 'cm' }, status: 'A' } ]);
$db->coll("inventory")->insert_many( [ { item => "canvas", qty => 100, size => { h => 28, w => 35.5, uom => "cm" }, status => "A" }, { item => "journal", qty => 25, size => { h => 14, w => 21, uom => "cm" }, status => "A" }, { item => "mat", qty => 85, size => { h => 27.9, w => 35.5, uom => "cm" }, status => "A" }, { item => "mousepad", qty => 25, size => { h => 19, w => 22.85, uom => "cm" }, status => "P" }, { item => "notebook", qty => 50, size => { h => 8.5, w => 11, uom => "in" }, status => "P" }, { item => "paper", qty => 100, size => { h => 8.5, w => 11, uom => "in" }, status => "D" }, { item => "planner", qty => 75, size => { h => 22.85, w => 30, uom => "cm" }, status => "D" }, { item => "postcard", qty => 45, size => { h => 10, w => 15.25, uom => "cm" }, status => "A" }, { item => "sketchbook", qty => 80, size => { h => 14, w => 21, uom => "cm" }, status => "A" }, { item => "sketch pad", qty => 95, size => { h => 22.85, w => 30.5, uom => "cm" }, status => "A" } ] );
$insertManyResult = $db->inventory->insertMany([ [ 'item' => 'canvas', 'qty' => 100, 'size' => ['h' => 28, 'w' => 35.5, 'uom' => 'cm'], 'status' => 'A', ], [ 'item' => 'journal', 'qty' => 25, 'size' => ['h' => 14, 'w' => 21, 'uom' => 'cm'], 'status' => 'A', ], [ 'item' => 'mat', 'qty' => 85, 'size' => ['h' => 27.9, 'w' => 35.5, 'uom' => 'cm'], 'status' => 'A', ], [ 'item' => 'mousepad', 'qty' => 25, 'size' => ['h' => 19, 'w' => 22.85, 'uom' => 'cm'], 'status' => 'P', ], [ 'item' => 'notebook', 'qty' => 50, 'size' => ['h' => 8.5, 'w' => 11, 'uom' => 'in'], 'status' => 'P', ], [ 'item' => 'paper', 'qty' => 100, 'size' => ['h' => 8.5, 'w' => 11, 'uom' => 'in'], 'status' => 'D', ], [ 'item' => 'planner', 'qty' => 75, 'size' => ['h' => 22.85, 'w' => 30, 'uom' => 'cm'], 'status' => 'D', ], [ 'item' => 'postcard', 'qty' => 45, 'size' => ['h' => 10, 'w' => 15.25, 'uom' => 'cm'], 'status' => 'A', ], [ 'item' => 'sketchbook', 'qty' => 80, 'size' => ['h' => 14, 'w' => 21, 'uom' => 'cm'], 'status' => 'A', ], [ 'item' => 'sketch pad', 'qty' => 95, 'size' => ['h' => 22.85, 'w' => 30.5, 'uom' => 'cm'], 'status' => 'A', ], ]);
db.inventory.insert_many( [ { "item": "canvas", "qty": 100, "size": {"h": 28, "w": 35.5, "uom": "cm"}, "status": "A", }, { "item": "journal", "qty": 25, "size": {"h": 14, "w": 21, "uom": "cm"}, "status": "A", }, { "item": "mat", "qty": 85, "size": {"h": 27.9, "w": 35.5, "uom": "cm"}, "status": "A", }, { "item": "mousepad", "qty": 25, "size": {"h": 19, "w": 22.85, "uom": "cm"}, "status": "P", }, { "item": "notebook", "qty": 50, "size": {"h": 8.5, "w": 11, "uom": "in"}, "status": "P", }, { "item": "paper", "qty": 100, "size": {"h": 8.5, "w": 11, "uom": "in"}, "status": "D", }, { "item": "planner", "qty": 75, "size": {"h": 22.85, "w": 30, "uom": "cm"}, "status": "D", }, { "item": "postcard", "qty": 45, "size": {"h": 10, "w": 15.25, "uom": "cm"}, "status": "A", }, { "item": "sketchbook", "qty": 80, "size": {"h": 14, "w": 21, "uom": "cm"}, "status": "A", }, { "item": "sketch pad", "qty": 95, "size": {"h": 22.85, "w": 30.5, "uom": "cm"}, "status": "A", }, ] )
client[:inventory].insert_many([ { item: 'canvas', qty: 100, size: { h: 28, w: 35.5, uom: 'cm' }, status: 'A' }, { item: 'journal', qty: 25, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }, { item: 'mat', qty: 85, size: { h: 27.9, w: 35.5, uom: 'cm' }, status: 'A' }, { item: 'mousepad', qty: 25, size: { h: 19, w: 22.85, uom: 'cm' }, status: 'P' }, { item: 'notebook', qty: 50, size: { h: 8.5, w: 11, uom: 'in' }, status: 'P' }, { item: 'paper', qty: 100, size: { h: 8.5, w: 11, uom: 'in' }, status: 'D' }, { item: 'planner', qty: 75, size: { h: 22.85, w: 30, uom: 'cm' }, status: 'D' }, { item: 'postcard', qty: 45, size: { h: 10, w: 15.25, uom: 'cm' }, status: 'A' }, { item: 'sketchbook', qty: 80, size: { h: 14, w: 21, uom: 'cm' }, status: 'A' }, { item: 'sketch pad', qty: 95, size: { h: 22.85, w: 30.5, uom: 'cm' }, status: 'A' } ])
collection.insertMany(Seq( Document("""{ item: "canvas", qty: 100, size: { h: 28, w: 35.5, uom: "cm" }, status: "A" }"""), Document("""{ item: "journal", qty: 25, size: { h: 14, w: 21, uom: "cm" }, status: "A" }"""), Document("""{ item: "mat", qty: 85, size: { h: 27.9, w: 35.5, uom: "cm" }, status: "A" }"""), Document("""{ item: "mousepad", qty: 25, size: { h: 19, w: 22.85, uom: "cm" }, status: "P" }"""), Document("""{ item: "notebook", qty: 50, size: { h: 8.5, w: 11, uom: "in" }, status: "P" }"""), Document("""{ item: "paper", qty: 100, size: { h: 8.5, w: 11, uom: "in" }, status: "D" }"""), Document("""{ item: "planner", qty: 75, size: { h: 22.85, w: 30, uom: "cm" }, status: "D" }"""), Document("""{ item: "postcard", qty: 45, size: { h: 10, w: 15.25, uom: "cm" }, status: "A" }"""), Document("""{ item: "sketchbook", qty: 80, size: { h: 14, w: 21, uom: "cm" }, status: "A" }"""), Document("""{ item: "sketch pad", qty: 95, size: { h: 22.85, w: 30.5, uom: "cm" }, status: "A" }""") )).execute()
Update Documents in a Collection
To update a document, MongoDB provides
update operators, such
as $set
, to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator>: { <field1>: <value1>, ... }, <update operator>: { <field2>: <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document in Compass, hover over the target document and click the pencil icon:
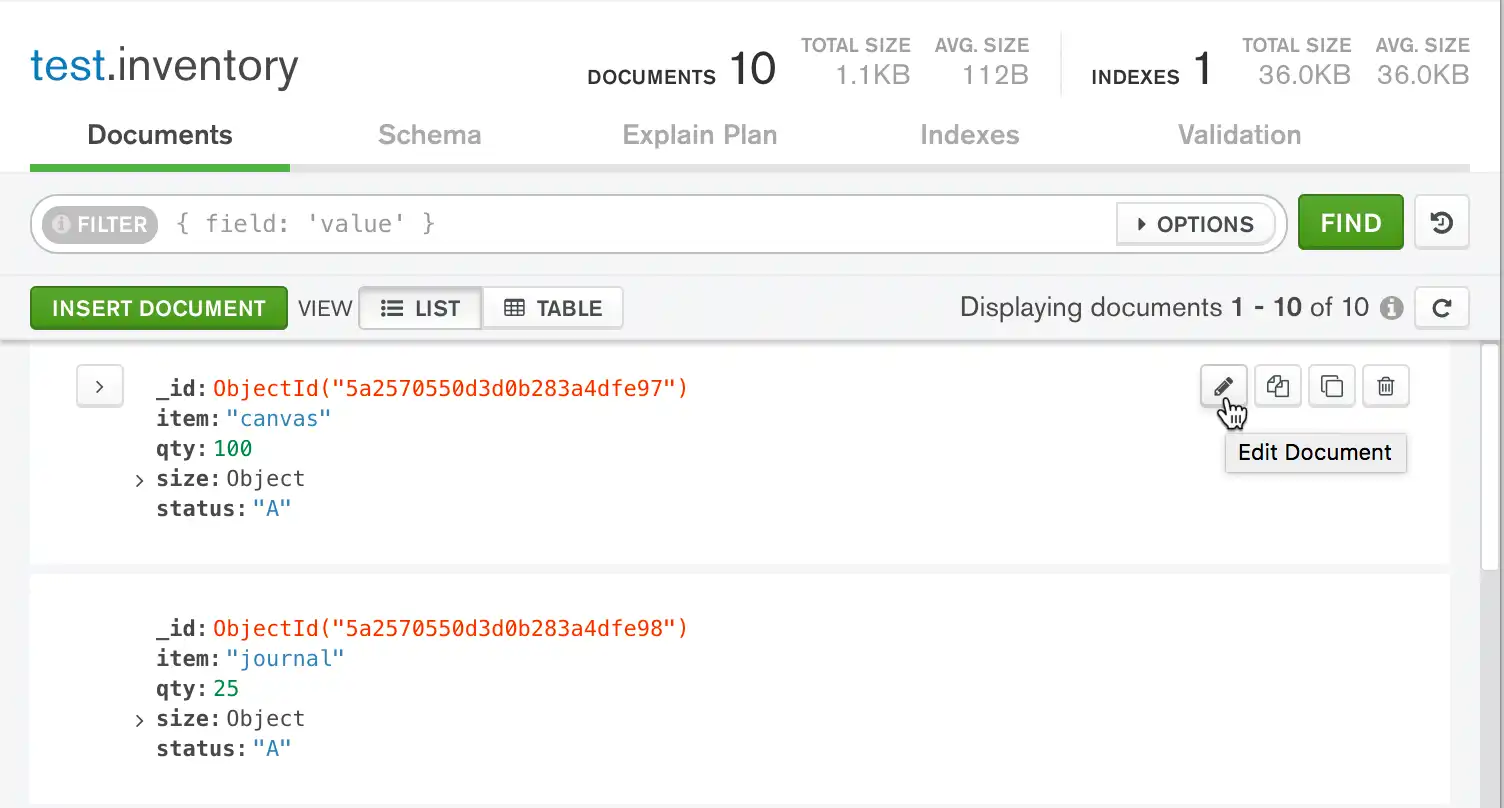
After clicking the pencil icon, the document enters edit mode:
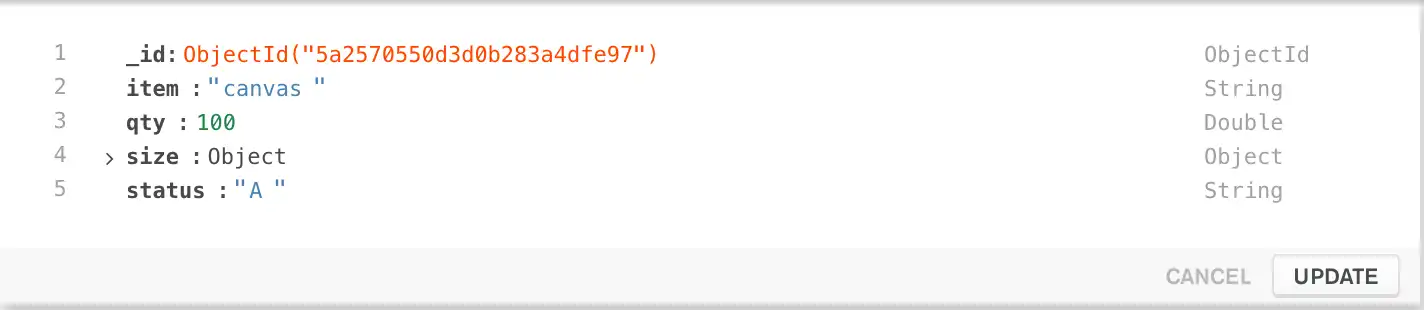
You can now change the this document by clicking the item you wish to change and modifying the value.
For detailed instructions on updating documents in Compass, refer to the Compass documentation or follow the example below.
Once you are satisfied with your changes, click Update to save the updated document.
Click Cancel to revert any modifications made to the document and exit edit mode.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator> => { <field1> => <value1>, ... }, <update operator> => { <field2> => <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
The driver provides the com.mongodb.client.model.Updates class to facilitate the creation of update documents. For example:
combine(set( <field1>, <value1>), set(<field2>, <value2> ) )
For a list of the update helpers, see com.mongodb.client.model.Updates.
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
The driver provides the com.mongodb.client.model.Updates class to facilitate the creation of update documents. For example:
combine(set( <field1>, <value1>), set(<field2>, <value2> ) )
For a list of the update helpers, see com.mongodb.client.model.Updates.
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator>: { <field1>: <value1>, ... }, <update operator>: { <field2>: <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator>: { <field1>: <value1>, ... }, <update operator>: { <field2>: <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator> => { <field1> => <value1>, ... }, <update operator> => { <field2> => <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
[ <update operator> => [ <field1> => <value1>, ... ], <update operator> => [ <field2> => <value2>, ... ], ... ]
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator>: { <field1>: <value1>, ... }, <update operator>: { <field2>: <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
{ <update operator> => { <field1> => <value1>, ... }, <update operator> => { <field2> => <value2>, ... }, ... }
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
To update a document, MongoDB provides
update operators such
as $set
to modify field values.
To use the update operators, pass to the update methods an update document of the form:
( set (<field1>, <value1>), set (<field2>, <value2>), ... )
Some update operators, such as $set
, will create the field if
the field does not exist. See the individual
update operator reference for
details.
Note
Starting in MongoDB 4.2, MongoDB can accept an aggregation pipeline to specify the modifications to make instead of an update document. See the method reference page for details.
Update a Single Document
The following example uses the
db.collection.updateOne()
method on the
inventory
collection to update the first document where
item
equals "paper"
:
The following example demonstrates using MongoDB Compass to modify
a single document where item: paper
in the inventory
collection:
Note
This example uses the Compass Table View to modify the document. The editing process using the Compass List View follows a very similar approach.
For more information on the differences between Table View and List View in Compass, refer to the Compass documentation.
The following example uses the
IMongoCollection.UpdateOne() method on the
inventory
collection to update the first document where
item
equals "paper"
:
The following example uses the
Collection.UpdateOne method
on the inventory
collection to update the first document
where item
equals "paper"
:
The following example uses the
com.mongodb.reactivestreams.client.MongoCollection.updateOne
on the inventory
collection to update the first document
where item
equals "paper"
:
The following example uses the
com.mongodb.client.MongoCollection.updateOne method on the
inventory
collection to update the first document where
item
equals "paper"
:
The following example uses the
update_one
method on the inventory
collection to update the first
document where item
equals "paper"
:
The following example uses the
Collection.updateOne()
method on the inventory
collection to update the first
document where item
equals "paper"
:
The following example uses the
update_one() method on the
inventory
collection to update the first document where
item
equals "paper"
:
The following example uses the updateOne()
method on the
inventory
collection to update the first document where
item
equals "paper"
:
The following example uses the
update_one
method on
the inventory
collection to update the first document
where item
equals "paper"
:
The following example uses the
update_one()
method on the inventory
collection to update the first
document where item
equals "paper"
:
The following example uses the
updateOne()
method on the inventory
collection to update the first
document where item
equals "paper"
:
db.inventory.updateOne( { item: "paper" }, { $set: { "size.uom": "cm", status: "P" }, $currentDate: { lastModified: true } } )
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
Modify the target document as follows:
Change the
status
field fromD
toP
.Change the
size.uom
field fromin
tocm
.Add a new field called
lastModified
whose value will be today's date.
Click the Table button in the top navigation to access the Table View:
Use the Compass query bar to locate the target document.
Copy the following filter document into the query bar and click Find:
{ item: "paper" } Hover over the
status
field and click the pencil icon which appears on the right side of the document to enter edit mode:Change the value of the field to
"P"
.Click the Update button below the field to save your changes.
Hover over the
size
field and click the outward-pointing arrows which appear on the right side of the field. This opens a new tab which displays the fields within thesize
object:Using the same process outlined in steps 3-5 for editing the
status
field, change the value of thesize.uom
field to"cm"
.Click the left-most tab above the table labelled
inventory
to return to the original table view, which displays the top-level document:Hover over the
status
field and click the pencil icon which appears on the right side of the document to re-enter edit mode.Click inside of the
status
field and click the plus button icon which appears in the edit menu.Click the Add Field After status button which appears below the plus button:
Add a new field called
lastModified
with a value of today's date. Set the field type toDate
:Click the Update button below the field to save your changes.
Note
Because MongoDB Compass does not support
$currentDate
or any other Field Update Operators, you must manually enter the date value in Compass.
var filter = Builders<BsonDocument>.Filter.Eq("item", "paper"); var update = Builders<BsonDocument>.Update.Set("size.uom", "cm").Set("status", "P").CurrentDate("lastModified"); var result = collection.UpdateOne(filter, update);
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
result, err := coll.UpdateOne( context.TODO(), bson.D{ {"item", "paper"}, }, bson.D{ {"$set", bson.D{ {"size.uom", "cm"}, {"status", "P"}, }}, {"$currentDate", bson.D{ {"lastModified", true}, }}, }, )
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
Publisher<UpdateResult> updateOnePublisher = collection.updateOne(eq("item", "paper"), combine(set("size.uom", "cm"), set("status", "P"), currentDate("lastModified")));
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
collection.updateOne(eq("item", "paper"), combine(set("size.uom", "cm"), set("status", "P"), currentDate("lastModified")));
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
await db.inventory.update_one( {"item": "paper"}, {"$set": {"size.uom": "cm", "status": "P"}, "$currentDate": {"lastModified": True}}, )
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
await db.collection('inventory').updateOne( { item: 'paper' }, { $set: { 'size.uom': 'cm', status: 'P' }, $currentDate: { lastModified: true } } );
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
# For boolean values, use boolean.pm for 'true' and 'false' $db->coll("inventory")->update_one( { item => "paper" }, { '$set' => { "size.uom" => "cm", status => "P" }, '$currentDate' => { lastModified => true } } );
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
$updateResult = $db->inventory->updateOne( ['item' => 'paper'], [ '$set' => ['size.uom' => 'cm', 'status' => 'P'], '$currentDate' => ['lastModified' => true], ], );
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
db.inventory.update_one( {"item": "paper"}, {"$set": {"size.uom": "cm", "status": "P"}, "$currentDate": {"lastModified": True}}, )
client[:inventory].update_one({ item: 'paper'}, { '$set' => { 'size.uom' => 'cm', 'status' => 'P' }, '$currentDate' => { 'lastModified' => true } })
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
collection.updateOne(equal("item", "paper"), combine(set("size.uom", "cm"), set("status", "P"), currentDate("lastModified")) ).execute()
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"cm"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
Update Multiple Documents
New in version 3.2.
The following example uses the
db.collection.updateMany()
method on the inventory
collection to update all documents where qty
is less than
50
:
You can update only one document at a time in MongoDB Compass.
To update multiple documents, connect to your
MongoDB deployment from mongo
or a MongoDB driver
and follow the examples in this section for your preferred method.
The following example uses the
IMongoCollection.UpdateMany() method on the
inventory
collection to update all documents where qty
is
less than 50
:
The following example uses the
Collection.UpdateMany
method on the inventory
collection to update all documents
where qty
is less than 50
:
The following example uses the
com.mongodb.reactivestreams.client.MongoCollection.updateMany
method on the inventory
collection to update all documents
where qty
is less than 50
:
The following example uses the
com.mongodb.client.MongoCollection.updateMany method on
the inventory
collection to update all documents where
qty
is less than 50
:
New in version 3.2.
The following example uses the
update_many
method on the inventory
collection to update all documents
where qty
is less than 50
:
The following example uses the Collection.updateMany() method on the inventory
collection to update all documents where qty
is less than
50
:
The following example uses the
update_many() method on the
inventory
collection to update all documents where qty
is
less than 50
:
The following example uses the updateMany()
method on the
inventory
collection to update all documents where qty
is
less than 50
:
New in version 3.2.
The following example uses the
update_many
method on
the inventory
collection to update all documents where qty
is less than 50
:
The following example uses the
update_many()
method on the inventory
collection to update all documents
where qty
is less than 50
:
The following example uses the
updateMany()
method on the inventory
collection to update all documents
where qty
is less than 50
:
db.inventory.updateMany( { "qty": { $lt: 50 } }, { $set: { "size.uom": "in", status: "P" }, $currentDate: { lastModified: true } } )
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
var filter = Builders<BsonDocument>.Filter.Lt("qty", 50); var update = Builders<BsonDocument>.Update.Set("size.uom", "in").Set("status", "P").CurrentDate("lastModified"); var result = collection.UpdateMany(filter, update);
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
result, err := coll.UpdateMany( context.TODO(), bson.D{ {"qty", bson.D{ {"$lt", 50}, }}, }, bson.D{ {"$set", bson.D{ {"size.uom", "cm"}, {"status", "P"}, }}, {"$currentDate", bson.D{ {"lastModified", true}, }}, }, )
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
Publisher<UpdateResult> updateManyPublisher = collection.updateMany(lt("qty", 50), combine(set("size.uom", "in"), set("status", "P"), currentDate("lastModified")));
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
collection.updateMany(lt("qty", 50), combine(set("size.uom", "in"), set("status", "P"), currentDate("lastModified")));
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
await db.inventory.update_many( {"qty": {"$lt": 50}}, {"$set": {"size.uom": "in", "status": "P"}, "$currentDate": {"lastModified": True}}, )
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
await db.collection('inventory').updateMany( { qty: { $lt: 50 } }, { $set: { 'size.uom': 'in', status: 'P' }, $currentDate: { lastModified: true } } );
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
# For boolean values, use boolean.pm for 'true' and 'false' $db->coll("inventory")->update_many( { qty => { '$lt' => 50 } }, { '$set' => { "size.uom" => "in", status => "P" }, '$currentDate' => { lastModified => true } } );
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
$updateResult = $db->inventory->updateMany( ['qty' => ['$lt' => 50]], [ '$set' => ['size.uom' => 'cm', 'status' => 'P'], '$currentDate' => ['lastModified' => true], ], );
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
db.inventory.update_many( {"qty": {"$lt": 50}}, {"$set": {"size.uom": "in", "status": "P"}, "$currentDate": {"lastModified": True}}, )
client[:inventory].update_many({ qty: { '$lt' => 50 } }, { '$set' => { 'size.uom' => 'in', 'status' => 'P' }, '$currentDate' => { 'lastModified' => true } })
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
collection.updateMany(lt("qty", 50), combine(set("size.uom", "in"), set("status", "P"), currentDate("lastModified")) ).execute()
The update operation:
uses the
$set
operator to update the value of thesize.uom
field to"in"
and the value of thestatus
field to"P"
,uses the
$currentDate
operator to update the value of thelastModified
field to the current date. IflastModified
field does not exist,$currentDate
will create the field. See$currentDate
for details.
Replace a Document
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
db.collection.replaceOne()
.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
You can't replace a document in MongoDB Compass.
To replace a document, connect to your
MongoDB deployment from mongo
or a MongoDB driver
and follow the examples in this section for your preferred method.
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
IMongoCollection.ReplaceOne().
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
Collection.ReplaceOne.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
com.mongodb.reactivestreams.client.MongoCollection.replaceOne.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
com.mongodb.client.MongoCollection.replaceOne.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
replace_one
.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the
_id
field, pass an entirely new document as the second
argument to
Collection.replaceOne().
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
replace_one().
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
replaceOne()
.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
replace_one
.
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
replace_one().
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
To replace the entire content of a document except for the _id
field, pass an entirely new document as the second argument to
replaceOne()
When replacing a document, the replacement document must consist of only field/value pairs; i.e. do not include update operators expressions.
The replacement document can have different fields from the original
document. In the replacement document, you can omit the _id
field
since the _id
field is immutable; however, if you do include the
_id
field, it must have the same value as the current value.
The following example replaces the first document from the
inventory
collection where item: "paper"
:
db.inventory.replaceOne( { item: "paper" }, { item: "paper", instock: [ { warehouse: "A", qty: 60 }, { warehouse: "B", qty: 40 } ] } )
var filter = Builders<BsonDocument>.Filter.Eq("item", "paper"); var replacement = new BsonDocument { { "item", "paper" }, { "instock", new BsonArray { new BsonDocument { { "warehouse", "A" }, { "qty", 60 } }, new BsonDocument { { "warehouse", "B" }, { "qty", 40 } } } } }; var result = collection.ReplaceOne(filter, replacement);
result, err := coll.ReplaceOne( context.TODO(), bson.D{ {"item", "paper"}, }, bson.D{ {"item", "paper"}, {"instock", bson.A{ bson.D{ {"warehouse", "A"}, {"qty", 60}, }, bson.D{ {"warehouse", "B"}, {"qty", 40}, }, }}, }, )
Publisher<UpdateResult> replaceOnePublisher = collection.replaceOne(eq("item", "paper"), Document.parse("{ item: 'paper', instock: [ { warehouse: 'A', qty: 60 }, { warehouse: 'B', qty: 40 } ] }"));
collection.replaceOne(eq("item", "paper"), Document.parse("{ item: 'paper', instock: [ { warehouse: 'A', qty: 60 }, { warehouse: 'B', qty: 40 } ] }"));
await db.inventory.replace_one( {"item": "paper"}, { "item": "paper", "instock": [{"warehouse": "A", "qty": 60}, {"warehouse": "B", "qty": 40}], }, )
await db.collection('inventory').replaceOne( { item: 'paper' }, { item: 'paper', instock: [ { warehouse: 'A', qty: 60 }, { warehouse: 'B', qty: 40 } ] } );
$db->coll("inventory")->replace_one( { item => "paper" }, { item => "paper", instock => [ { warehouse => "A", qty => 60 }, { warehouse => "B", qty => 40 } ] } );
$updateResult = $db->inventory->replaceOne( ['item' => 'paper'], [ 'item' => 'paper', 'instock' => [ ['warehouse' => 'A', 'qty' => 60], ['warehouse' => 'B', 'qty' => 40], ], ], );
db.inventory.replace_one( {"item": "paper"}, { "item": "paper", "instock": [{"warehouse": "A", "qty": 60}, {"warehouse": "B", "qty": 40}], }, )
client[:inventory].replace_one({ item: 'paper' }, { item: 'paper', instock: [ { warehouse: 'A', qty: 60 }, { warehouse: 'B', qty: 40 } ] })
collection.replaceOne(equal("item", "paper"), Document("""{ item: "paper", instock: [ { warehouse: "A", qty: 60 }, { warehouse: "B", qty: 40 } ] }""") ).execute()
Update a Document with MongoDB Atlas
Note
You can update only one document at a time in the MongoDB Atlas UI.
To update multiple documents or replace an entire document,
connect to your Atlas deployment from mongo
or a MongoDB driver and follow the examples on this page
for your preferred method.
The example in this section uses the sample supplies dataset. To learn how to load the sample dataset into your MongoDB Atlas deployment, see Load Sample Data.
To update a document in MongoDB Atlas, follow these steps:
Specify a query filter document.
You can specify a query filter document in the Filter field. A query filter document uses query operators to specify search conditions.
Copy the following query filter document into the Filter search bar and click Apply:
{ saleDate: { $gte: { $date: "2016-01-01T00:00-00:00" }, $lte: { $date: "2016-01-02T00:00-00:00" } } }
This query filter returns all documents in the sample_supplies.sales
collection where saleDate
is on or between January 1 and 2, 2016
UTC time.
Edit a document.
To edit a document displayed in the query results, hover over the document and click on the pencil icon. In the document editor, you can:
Add a new field.
Delete an existing field.
Edit a field's name, value, or type.
Revert a specific change.
For detailed instructions, see Create, View, Update, and Delete Documents.
Behavior
Atomicity
All write operations in MongoDB are atomic on the level of a single document. For more information on MongoDB and atomicity, see Atomicity and Transactions.
_id
Field
Once set, you cannot update the value of the _id
field nor can you
replace an existing document with a replacement document that has a
different _id
field value.
Field Order
For write operations, MongoDB preserves the order of the document fields except for the following cases:
The
_id
field is always the first field in the document.Updates that include
renaming
of field names may result in the reordering of fields in the document.
Upsert Option
If updateOne()
,
updateMany()
, or
replaceOne()
includes upsert : true
and no documents match the specified filter, then the
operation creates a new document and inserts it. If there are
matching documents, then the operation modifies or replaces the
matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
The upsert option is not available through MongoDB Compass.
If UpdateOne(),
UpdateMany(), or
ReplaceOne() includes an
UpdateOptions
argument instance with the IsUpsert
option set to true
and no documents match the specified filter, then the
operation creates a new document and inserts it. If there are
matching documents, then the operation modifies or replaces the
matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If Collection.UpdateOne includes the Upsert option set to true and no documents match the specified filter, then the operation creates a new document and inserts it. If there are matching documents, then the operation modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If the update and replace methods include the UpdateOptions parameter that specifies UpdateOptions.upsert(true) and no documents match the specified filter, then the operation creates a new document and inserts it. If there are matching documents, then the operation modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If the update and replace methods include the com.mongodb.client.model.UpdateOptions parameter that specifies com.mongodb.client.model.UpdateOptions.upsert(true) and no documents match the specified filter, then the operation creates a new document and inserts it. If there are matching documents, then the operation modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If
update_one
,
update_many
,
or
replace_one
includes upsert : true
and no documents match the
specified filter, then the operation creates a new document and
inserts it. If there are matching documents, then the operation
modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If updateOne(),
updateMany(), or
replaceOne() include
upsert : true
in the options
parameter document and
no documents match the specified filter, then the operation
creates a new document and inserts it. If there are matching
documents, then the operation modifies or replaces the matching
document or documents.
For details on the new document created, see the individual reference pages for the methods.
If update_one(),
update_many(), or
replace_one() includes
upsert => true
and no documents match the specified
filter, then the operation creates a new document and inserts
it. If there are matching documents, then the operation
modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If updateOne()
,
updateMany()
, or
replaceOne()
includes upsert =>
true
and no documents match the specified filter, then the
operation creates a new document and inserts it. If there are
matching documents, then the operation modifies or replaces the
matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If update_one
,
update_many
, or
replace_one
includes
upsert : true
and no documents match the specified
filter, then the operation creates a new document and inserts
it. If there are matching documents, then the operation
modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If
update_one(),
update_many(),
or
replace_one()
includes upsert => true
and no documents match the
specified filter, then the operation creates a new document and
inserts it. If there are matching documents, then the operation
modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
If
updateOne(),
updateMany(),
or
replaceOne()
includes upsert => true
and no documents match the
specified filter, then the operation creates a new document and
inserts it. If there are matching documents, then the operation
modifies or replaces the matching document or documents.
For details on the new document created, see the individual reference pages for the methods.
Write Acknowledgement
With write concerns, you can specify the level of acknowledgement requested from MongoDB for write operations. For details, see Write Concern.